בדיקת פרונט-אנד של ראקט עם ינון פרק
טסטינג ראקט
- ToCode
- ToCode blog
- ינון פרק
- React Testing Library
- Kutt
- Kutt Github repo
- Fork of Ynon
- Tests by Ynon
- Jest test fails : TypeError: window.matchMedia is not a function
- ערוץ יוטוב של מביני קוד
- דף לינקדאין של מביני קוד
examples/footer_test.tsx
import React from "react"; import { render } from "@testing-library/react"; import { StoreProvider } from "easy-peasy"; import { initializeStore } from "../../store"; import Footer from "../Footer"; import getConfig from "next/config"; describe("<Footer /> component test", () => { let app; beforeEach(() => { const store = initializeStore(); app = ( <StoreProvider store={store}> <Footer /> </StoreProvider> ); }); it("should contain a github link", () => { const screen = render(app); const githubLink = screen.getByRole("link", { name: "GitHub" }); expect(githubLink).toHaveAttribute("href", "https://github.com/thedevs-network/kutt"); }); it("should contain a TOS link", () => { const config = getConfig(); const screen = render(app); const tosLink = screen.getByRole("link", { name: "Terms of Service" }); expect(tosLink).toHaveAttribute("href", "/terms"); }); it("should show contact email if defined", () => { const config = getConfig(); config.publicRuntimeConfig.CONTACT_EMAIL = 'foobar'; const screen = render(app); const emailLink = screen.getByRole("link", { name: "Contact us" }); expect(emailLink).toHaveAttribute("href", "mailto:foobar"); }); it("should NOT show contact email if none is defined", () => { const config = getConfig(); delete(config.publicRuntimeConfig.CONTACT_EMAIL); const screen = render(app); const emailLink= screen.queryByRole("link", { name: "Contact us" }); expect(emailLink).toBeNull(); }); })
examples/shortener_test.tsx
import React from "react"; import { render } from "@testing-library/react"; import { StoreProvider, createStore, Thunk, thunk } from "easy-peasy"; import userEvent from "@testing-library/user-event" import { store } from "../../store"; import { links, Link, Links, NewLink } from "../../store/links"; import Shortener from "../Shortener"; describe("<Shortener /> component test", () => { let app; beforeEach(() => { store.links = { ...store.links, submit: thunk(async (actions, payload) => { return { id: "0", address: "localhost:3000/foobar", banned: false, created_at: "now", link: "localhost:3000/foobar", target: "", updated_at: "now", visit_count: 0 }; }) }; const testStore = createStore(store); app = ( <StoreProvider store={testStore}> <Shortener /> </StoreProvider> ); }); it("Should not sumbit an empty string", async () => { const screen = render(app); const urlInput = screen.getByRole("textbox", { name: "target" }); const submitButton = screen.getByRole("button", { name: "submit" }); userEvent.click(submitButton); const msg = await screen.findByText(/Missing target URL/i); expect(msg).toBeInTheDocument(); }); it("Should show the short URL", async () => { const screen = render(app); const urlInput = screen.getByRole("textbox", { name: "target" }); userEvent.type(urlInput, "https://easy-peasy.now.sh/docs/api/thunk.html"); const submitButton = screen.getByRole("button", { name: "submit" }); userEvent.click(submitButton); const msg = await screen.findByText(/localhost:3000\/foobar/i); expect(msg).toBeInTheDocument(); }); it("Should empty target input", async () => { const screen = render(app); let urlInput: HTMLInputElement = screen.getByRole("textbox", { name: "target" }) as HTMLInputElement; userEvent.type(urlInput, "https://easy-peasy.now.sh/docs/api/thunk.html"); const submitButton = screen.getByRole("button", { name: "submit" }); userEvent.click(submitButton); const msg = await screen.findByText(/localhost:3000\/foobar/i); urlInput = screen.getByRole("textbox", { name: "target" }) as HTMLInputElement; expect(urlInput.value).toEqual(""); }); });
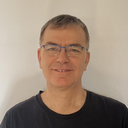
Published on 2021-03-12
במידה ויש לכם תגובות או שאלות לגבי דף זה, הרגישו חופשי להעלות אותם בעמוד זה בגיטהאב מקור בגיטהאב
Comment on this post